この記事で得られること
今回はバトルシステムの実装を紹介します。
特にUIを中心に作っていきます。ざっくり下のような配置にします。
(基本的には動画の流れを書いてるので、詳しいところは動画を見た方がいいです)
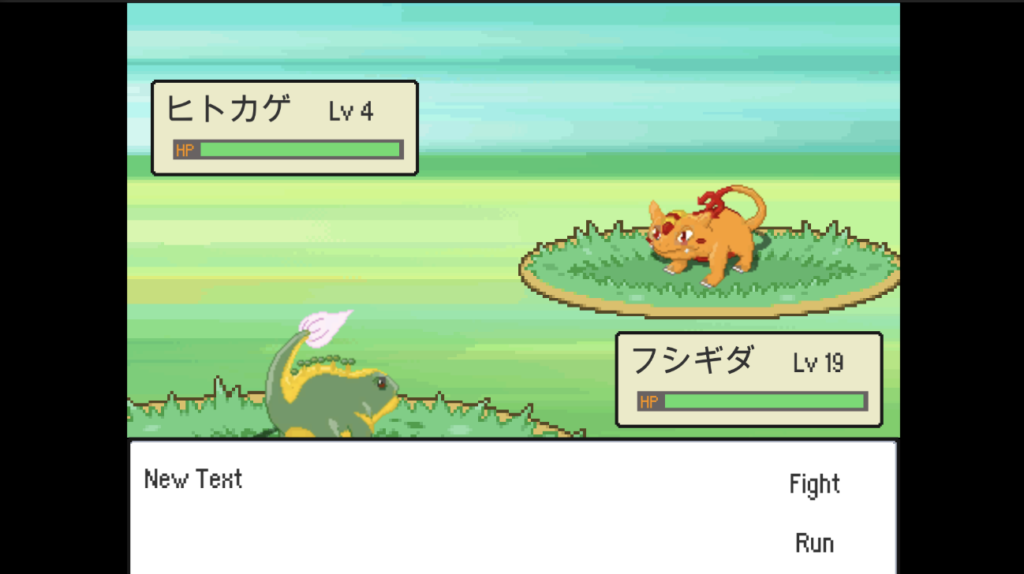
解説動画
Battle用のカメラとUIを作成
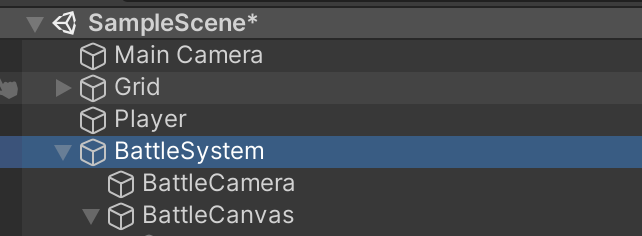
背景設定
メッセージボックス(DialogBox)の作成
この辺りは細かい設定をしてるから動画を参考に。
構成は
・背景画像
・メッセージのテキスト
・アクション(攻撃/逃げる)のテキスト
モンスター画像の設定
モンスターのディスプレイ作成
この辺りは細かい設定をしてるから動画を参考に。
構成は
・名前テキスト
・レベルテキスト
・HPバー
・背景
・HP
・テキスト
解説動画
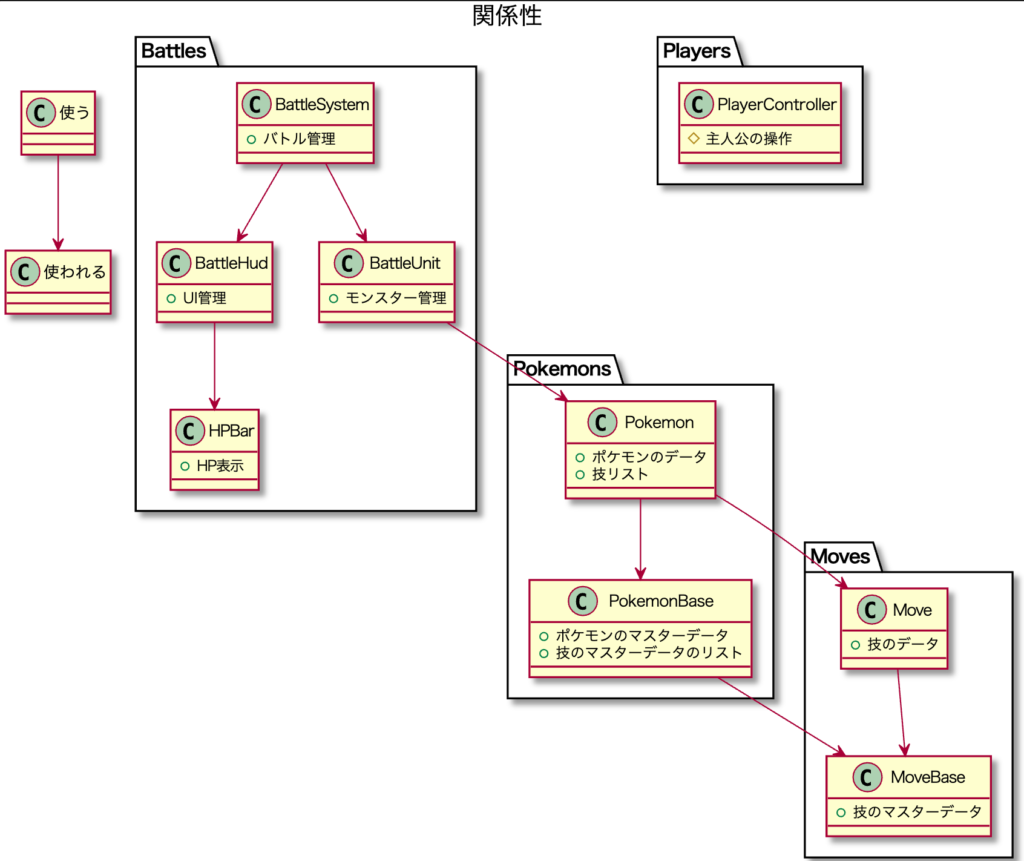
HPBarの実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HPBar : MonoBehaviour
{
[SerializeField] GameObject health;
public void SetHP(float hp)
{
health.transform.localScale = new Vector3(hp, 1, 1);
}
}
BattleHudの実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class BattleHud : MonoBehaviour
{
[SerializeField] Text nameText;
[SerializeField] Text levelText;
[SerializeField] HPBar hpBar;
public void SetData(Pokemon pokemon)
{
nameText.text = pokemon.Base.Name;
levelText.text = "Lv " + pokemon.Level;
hpBar.SetHP((float)pokemon.HP / pokemon.MaxHP);
}
}
修正:Pokemon.csでプロパティを作っておく
BattleUnitの作成
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class BattleUnit : MonoBehaviour
{
[SerializeField] PokemonBase _base;
[SerializeField] int level;
[SerializeField] bool isPlayerUnit;
public Pokemon Pokemon { get; set; }
public void Setup()
{
Pokemon = new Pokemon(_base, level);
Image image = GetComponent<Image>();
if (isPlayerUnit)
{
image.sprite = Pokemon.Base.BackSprite;
}
else
{
image.sprite = Pokemon.Base.FrontSprite;
}
}
}
BattleSystemの実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BattleSystem : MonoBehaviour
{
[SerializeField] BattleUnit playerUnit;
[SerializeField] BattleUnit enemyUnit;
[SerializeField] BattleHud playerHud;
[SerializeField] BattleHud enemyHud;
private void Start()
{
SetupBattle();
}
void SetupBattle()
{
playerUnit.Setup();
enemyUnit.Setup();
playerHud.SetData(playerUnit.Pokemon);
enemyHud.SetData(enemyUnit.Pokemon);
}
}
おさらいと予告
今回はバトルシステムのUIを作成しました。
質問対応について
動画質問はYouTubeのメンバーシップのDiscord(音声とチャット)で受け付けています。
個人開発のサポートはオンラインサロンで受け付けています!
コメント