この記事で得られること
今回は、モンスターの実装を紹介するぞ!
色々なモンスターを実装する必要があるので、ScriptableObjectを活用するぞ!
(基本的には動画の流れを書いてるので、詳しいところは動画を見た方がいいです)
解説動画
モンスターのScriptableObjectのコードを作成
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[CreateAssetMenu(fileName = "Pokemon", menuName = "Create New Pokemon")]
public class PokemonBase : ScriptableObject
{
[SerializeField] new string name;
// 説明
[TextArea]
[SerializeField] string description;
// 画像
[SerializeField] Sprite frontSprite;
[SerializeField] Sprite backSprite;
// タイプ1,2
[SerializeField] PokemonType type1;
[SerializeField] PokemonType type2;
[SerializeField] int maxHP;
[SerializeField] int attack;
[SerializeField] int defense;
[SerializeField] int spAttack; // 特攻
[SerializeField] int spDefense; // 特防御
[SerializeField] int speed;
}
public enum PokemonType
{
None, // なし
Fire, // 火
Water, // 水
Electric, // 電気
Grass, // 草
Ice, // 氷
Fighting, // 格闘
Poison, // 毒
Ground, // 地面
Flying, // 飛行
Psychic, // エスパー
Bug, // 虫
Rock, // 岩
Ghost, // ゴースト
Dragon, // ドラゴン
}
パラメータの反映
パラメータ参考:
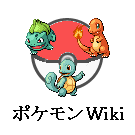
種族値一覧 (第六世代)
レベルに応じたモンスターClassの作成
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Pokemon
{
// レベルに応じて変化するステータスをもったポケモンクラス
PokemonBase _base;
int level;
public Pokemon(PokemonBase pBase, int pLevel)
{
_base = pBase;
level = pLevel;
}
public int Attack
{
get { return Mathf.FloorToInt((_base.Attack * level) / 100f) + 5; }
}
public int Defense
{
get { return Mathf.FloorToInt((_base.Defense * level) / 100f) + 5; }
}
public int SpAttack
{
get { return Mathf.FloorToInt((_base.SpAttack * level) / 100f) + 5; }
}
public int SpDefense
{
get { return Mathf.FloorToInt((_base.SpDefense * level) / 100f) + 5; }
}
public int Speed
{
get { return Mathf.FloorToInt((_base.Speed * level) / 100f) + 5; }
}
public int MaxHP
{
get { return Mathf.FloorToInt((_base.MaxHP * level) / 100f) + 10; }
}
}
おさらいと予告
今回はモンスターの多様化とレベルによる多様化を実装したぞ!
次は技の実装を行うぞ!
質問対応について
動画質問はYouTubeのメンバーシップのDiscord(音声とチャット)で受け付けています。
個人開発のサポートはオンラインサロンで受け付けています!
コメント